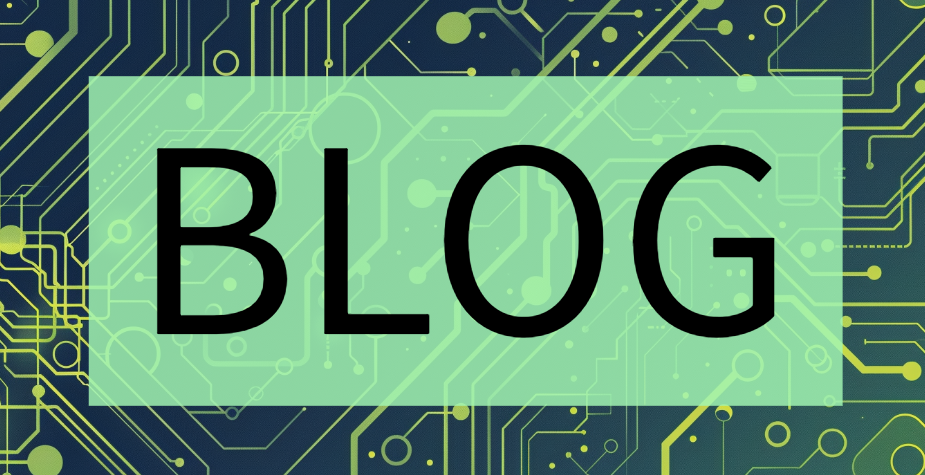
Getting Started with Data Analytics Using PyArrow in Python
- Apache Iceberg Crash Course: What is a Data Lakehouse and a Table Format?
- Free Copy of Apache Iceberg the Definitive Guide
- Free Apache Iceberg Crash Course
- Iceberg Lakehouse Engineering Video Playlist
Introduction
Overview of the Docker Environment
In this guide, we will explore data analytics using PyArrow, a powerful library designed for efficient in-memory data processing with columnar storage. We will work within a pre-configured environment using the Python Data Science Notebook Docker Image. This environment includes all the essential libraries for data manipulation, machine learning, and database connectivity, making it an ideal setup for performing analytics with PyArrow.
To get started, you can pull and run the Docker container by following these steps:
- Build the Docker Image:
docker pull alexmerced/datanotebook
Run the Container:
docker run -p 8888:8888 -v $(pwd):/home/pydata/work alexmerced/datanotebook
Access Jupyter Notebook: Open your browser and navigate to http://localhost:8888 to access the notebook interface.
This setup provides a user-friendly experience with Jupyter Notebook running on port 8888, where you can easily write and execute Python code for data analysis.
Why PyArrow?
Apache Arrow is an open-source framework optimized for in-memory data processing with a columnar format. PyArrow, the Python implementation of Arrow, enables faster, more efficient data access and manipulation compared to traditional column-based libraries like Pandas. Here are some key benefits of using PyArrow:
-
Faster Data Processing: PyArrow uses a columnar memory layout that accelerates access to large datasets.
-
Lower Memory Usage: Thanks to Arrow’s efficient memory format, you can handle larger datasets with less memory.
-
Interoperability: PyArrow integrates smoothly with other systems and languages, making it a versatile tool for multi-language environments.
-
Better Support for Large Datasets: PyArrow is designed to handle big data tasks, making it ideal for workloads that Pandas struggles with.
Section 1: Understanding Key PyArrow Objects
PyArrow provides a set of data structures that are specifically optimized for in-memory analytics and manipulation. In this section, we will explore the key objects in PyArrow and their purposes.
PyArrow’s Core Data Structures:
- Table:
- A
Table
in PyArrow is a collection of columnar data, optimized for efficient processing and memory usage. - It can be thought of as similar to a DataFrame in Pandas but designed to work seamlessly with Arrow’s columnar format.
- Tables can be partitioned and processed in parallel, which improves performance with large datasets.
- A
import pyarrow as pa
data = [
pa.array([1, 2, 3]),
pa.array(['A', 'B', 'C']),
]
table = pa.Table.from_arrays(data, names=['column1', 'column2'])
print(table)
- RecordBatch:
A RecordBatch is a collection of rows with a defined schema. It allows for efficient in-memory processing of data in batches.
It’s useful when you need to process data in chunks, enabling better memory management.
Example:
batch = pa.RecordBatch.from_pandas(df)
print(batch)
- Array:
An Array in PyArrow is a fundamental data structure representing a one-dimensional, homogeneous sequence of values.
Arrays can be of various types, including integers, floats, strings, and more. PyArrow provides specialized arrays for different types of data.
Example:
array = pa.array([1, 2, 3, 4, 5])
print(array)
- Schema:
A Schema defines the structure of data in a Table or RecordBatch. It consists of the names and data types of each column.
Schemas ensure that all data being processed follows a consistent format.
Example:
schema = pa.schema([
('column1', pa.int32()),
('column2', pa.string())
])
print(schema)
- ChunkedArray:
A ChunkedArray is a sequence of Array objects that have been split into smaller chunks. This allows for parallel processing on chunks of data, improving efficiency when working with larger datasets.
Example:
chunked_array = pa.chunked_array([[1, 2, 3], [4, 5, 6]])
print(chunked_array)
Summary
These core objects are essential for working with PyArrow and enable efficient data processing in memory. By utilizing PyArrow’s columnar format and its efficient handling of large datasets, you can perform complex data manipulations with ease. As we continue, you’ll see how these objects interact to make reading, writing, and analyzing data faster and more memory-efficient.
Section 2: Reading and Writing Parquet Files with PyArrow
Parquet is a columnar storage file format that is widely used in big data analytics. Its efficient compression and encoding make it ideal for storing large datasets. In this section, we will explore how to use PyArrow to read from and write to Parquet files.
Why Use Parquet?
- Efficient Storage: Parquet’s columnar format allows for efficient compression, reducing the storage size of large datasets.
- Faster Querying: By storing data in columns, Parquet files allow analytical queries to scan only the relevant columns, reducing I/O and improving performance.
- Interoperability: Parquet is a widely supported format that can be read and written by many different systems, making it ideal for data exchange.
Reading Parquet Files
Using PyArrow, you can easily read a Parquet file into memory as a PyArrow Table
. This table can then be used for further data processing or manipulation.
Example:
import pyarrow.parquet as pq
# Reading a Parquet file
table = pq.read_table('sample_data.parquet')
# Displaying the contents of the PyArrow table
print(table)
In this example, the pq.read_table()
function reads the Parquet file and returns a Table
object. This table can now be used for in-memory operations such as filtering, joining, or aggregating data.
Writing Parquet Files
To store data as Parquet, you can write a PyArrow Table back to disk in Parquet format. PyArrow provides methods for this purpose, allowing you to save your data efficiently.
Example:
import pyarrow as pa
import pyarrow.parquet as pq
# Create a simple PyArrow table
data = [
pa.array([1, 2, 3, 4]),
pa.array(['A', 'B', 'C', 'D'])
]
table = pa.Table.from_arrays(data, names=['column1', 'column2'])
# Writing the table to a Parquet file
pq.write_table(table, 'output_data.parquet')
In this example, a PyArrow table is created and saved to disk as a Parquet file using the pq.write_table()
function.
Working with Large Datasets
One of the key advantages of Parquet is its ability to handle large datasets efficiently. When reading a Parquet file, you can load only specific columns into memory, which is especially useful when working with large datasets.
Example:
# Reading only specific columns from a Parquet file
table = pq.read_table('sample_data.parquet', columns=['column1'])
print(table)
This code demonstrates how to read only the relevant columns, reducing the memory footprint when loading the dataset.
Summary
By using PyArrow to read and write Parquet files, you gain access to a highly efficient, compressed, and columnar data format that works well for large datasets. PyArrow simplifies working with Parquet by providing easy-to-use functions for loading and saving data, while also supporting advanced operations like selective column reads to optimize performance.
Section 3: Basic Analytical Operations with PyArrow
PyArrow not only provides efficient tools for reading and writing Parquet files but also enables you to perform basic data analytics operations like filtering, joining, and aggregating data in memory. These operations can be performed directly on PyArrow Table
objects, offering a significant performance boost when dealing with large datasets.
Filtering Data
PyArrow allows you to filter rows based on conditions, similar to how you would with Pandas. This operation is highly efficient due to the columnar nature of PyArrow’s data structures.
Example:
import pyarrow.compute as pc
# Assume we have a table with two columns: 'column1' and 'column2'
table = pq.read_table('sample_data.parquet')
# Apply a filter to keep rows where 'column1' > 2
filtered_table = table.filter(pc.greater(table['column1'], 2))
print(filtered_table)
In this example, we use PyArrow’s compute module to filter the data. The pc.greater() function returns a boolean mask, and the filter() method applies this mask to the table, returning only rows where ‘column1’ is greater than 2.
Joining Data
Just like in SQL or Pandas, PyArrow allows you to join two tables based on a common column. This operation is particularly useful when combining datasets.
Example:
import pyarrow as pa
# Creating two tables to join
left_table = pa.table({'key': [1, 2, 3], 'value_left': ['A', 'B', 'C']})
right_table = pa.table({'key': [1, 2, 3], 'value_right': ['X', 'Y', 'Z']})
# Performing an inner join on the 'key' column
joined_table = left_table.join(right_table, keys='key')
print(joined_table)
Here, we use PyArrow’s join method to perform an inner join on two tables, combining them based on the common column ‘key’. The result is a new table with data from both tables.
Aggregation Operations
Aggregation operations like summing, counting, and averaging are essential for data analytics. PyArrow provides efficient methods to perform these operations on large datasets.
Example:
import pyarrow.compute as pc
# Assume we have a table with a numerical column 'column1'
table = pq.read_table('sample_data.parquet')
# Perform aggregation: sum of 'column1'
sum_column1 = pc.sum(table['column1'])
print(f"Sum of column1: {sum_column1.as_py()}")
In this example, we use the pc.sum()
function to calculate the sum of a column. Similarly, you can apply other aggregation functions like pc.mean()
, pc.min()
, or pc.max()
.
Combining Operations: Filter and Aggregate
PyArrow allows you to chain operations together, such as filtering the data first and then applying aggregation.
Example:
# Filter the table where 'column1' > 2
filtered_table = table.filter(pc.greater(table['column1'], 2))
# Sum the filtered data in 'column1'
sum_filtered = pc.sum(filtered_table['column1'])
print(f"Sum of filtered column1: {sum_filtered.as_py()}")
In this case, we first filter the data and then apply the aggregation function on the filtered subset. This combination of operations enables more complex analyses with just a few lines of code.
Summary
PyArrow’s powerful analytical capabilities make it a great choice for performing data operations on large datasets. By leveraging its efficient in-memory structures, you can filter, join, and aggregate data in a way that is both fast and memory-efficient. Whether you are working with small or large datasets, PyArrow provides the tools to handle your data analytics tasks with ease.
Section 4: Working with JSON, CSV, and Feather Files using PyArrow
In addition to Parquet, PyArrow supports a wide variety of file formats, including JSON, CSV, and Feather. These formats are commonly used for data storage and interchange, and PyArrow makes it easy to read from and write to them efficiently.
Reading and Writing JSON Files
JSON (JavaScript Object Notation) is a lightweight data-interchange format that is widely used for data transfer. While it may not be as efficient as columnar formats like Parquet, JSON is still commonly used, especially for web data.
Reading JSON Files
PyArrow allows you to read JSON data and convert it into a PyArrow Table
for further processing.
Example:
import pyarrow.json as paj
# Reading a JSON file into a PyArrow table
table = paj.read_json('sample_data.json')
# Display the contents of the table
print(table)
Writing JSON Files
PyArrow can also write Table data back into JSON format, making it convenient for exchanging data in systems where JSON is the preferred format.
Example:
import pyarrow as pa
import pyarrow.json as paj
# Create a simple PyArrow table
data = {
'column1': [1, 2, 3],
'column2': ['A', 'B', 'C']
}
table = pa.Table.from_pydict(data)
# Writing the table to a JSON file
paj.write_json(table, 'output_data.json')
Reading and Writing CSV Files
CSV (Comma-Separated Values) is one of the most common file formats for structured data, particularly in data science and analytics. PyArrow makes it easy to work with CSV files by converting them to Table objects.
Reading CSV Files
PyArrow’s CSV reader allows for fast parsing of large CSV files, which can then be converted into tables for in-memory analytics.
Example:
import pyarrow.csv as pac
# Reading a CSV file into a PyArrow table
table = pac.read_csv('sample_data.csv')
# Display the table
print(table)
Writing CSV Files
You can also write PyArrow tables back to CSV format, which is helpful for data sharing and reporting.
Example:
import pyarrow as pa
import pyarrow.csv as pac
# Create a simple PyArrow table
data = {
'column1': [1, 2, 3],
'column2': ['A', 'B', 'C']
}
table = pa.Table.from_pydict(data)
# Writing the table to a CSV file
pac.write_csv(table, 'output_data.csv')
Reading and Writing Feather Files
Feather is a binary columnar file format that provides better performance compared to CSV and JSON, while maintaining interoperability between Python and R. PyArrow natively supports Feather, allowing for efficient storage and fast reads.
Reading Feather Files
Feather files are ideal for fast I/O operations and work seamlessly with PyArrow.
Example:
import pyarrow.feather as paf
# Reading a Feather file into a PyArrow table
table = paf.read_table('sample_data.feather')
# Display the table
print(table)
Writing Feather Files
PyArrow can write Table objects to Feather format, offering a balance between ease of use and performance, particularly for in-memory data sharing.
Example:
import pyarrow as pa
import pyarrow.feather as paf
# Create a simple PyArrow table
data = {
'column1': [1, 2, 3],
'column2': ['A', 'B', 'C']
}
table = pa.Table.from_pydict(data)
# Writing the table to a Feather file
paf.write_feather(table, 'output_data.feather')
Summary
PyArrow’s support for various file formats—such as JSON, CSV, and Feather—makes it a versatile tool for data analytics. Whether you’re working with structured CSVs, exchanging JSON data, or aiming for faster performance with Feather files, PyArrow simplifies the process of reading and writing these formats. This flexibility allows you to handle a wide range of data tasks, from data ingestion to efficient storage and retrieval.
Section 5: Using Apache Arrow Flight with PyArrow
Apache Arrow Flight is a high-performance data transport layer built on top of Apache Arrow. It provides an efficient way to transfer large datasets between systems. One of its key benefits is the ability to perform fast, scalable data transfers using gRPC for remote procedure calls. In this section, we will explore how to use Apache Arrow Flight with PyArrow with an example of connecting to Dremio, a popular data platform that supports Arrow Flight for query execution.
Connecting to Dremio Using PyArrow Flight
Below is an example of how to connect to Dremio using PyArrow Flight, execute a query, and retrieve the results.
from pyarrow import flight
from pyarrow.flight import FlightClient
import os
# Step 1: Set the location of the Arrow Flight server
location = "grpc+tls://data.dremio.cloud:443"
# Step 2: Obtain the authentication token (from environment variables in this case)
token = os.getenv("token")
# Step 3: Define the headers for the Flight requests
# Here, we pass the bearer token for authentication
headers = [
(b"authorization", f"bearer {token}".encode("utf-8"))
]
# Step 4: Write the SQL query you want to execute
query = "SELECT * FROM table1"
# Step 5: Create a FlightClient instance to connect to the server
client = FlightClient(location=location)
# Step 6: Set up FlightCallOptions to include the authorization headers
options = flight.FlightCallOptions(headers=headers)
# Step 7: Request information about the query's execution
flight_info = client.get_flight_info(flight.FlightDescriptor.for_command(query), options)
# Step 8: Fetch the results of the query
results = client.do_get(flight_info.endpoints[0].ticket, options)
# Step 9: Read and print the results from the server
print(results.read_all())
Explanation of Each Step
- Set the Flight Server Location:
location = "grpc+tls://data.dremio.cloud:443"
The location variable holds the address of the Dremio server that supports Apache Arrow Flight. Here, we use gRPC over TLS for a secure connection to Dremio Cloud.
- Authentication with Bearer Token:
token = os.getenv("token")
The token is retrieved from an environment variable using os.getenv()
. This token is required for authenticating requests to Dremio’s Arrow Flight server.
- Setting Request Headers:
headers = [
(b"authorization", f"bearer {token}".encode("utf-8"))
]
The headers include an authorization field with the bearer token, which is required for Dremio to authenticate the request. We use the FlightCallOptions
to attach this header to our request later.
- SQL Query:
query = "SELECT * FROM table1"
This is the SQL query we will execute on Dremio. You can replace “table1” with any table or a more complex SQL query as needed.
- Creating the FlightClient:
client = FlightClient(location=location)
The FlightClient
is the main object used to interact with the Arrow Flight server. It is initialized with the location of the server, allowing us to send requests and receive results.
- Setting Flight Call Options:
options = flight.FlightCallOptions(headers=headers)
Here, FlightCallOptions is used to attach the headers (including our authentication token) to the requests made by the FlightClient.
- Fetching Flight Information:
flight_info = client.get_flight_info(flight.FlightDescriptor.for_command(query), options)
The get_flight_info()
function sends the query to Dremio and returns information about the query’s execution, such as where the results are located. The FlightDescriptor.for_command()
method is used to wrap the SQL query into a format understood by the Flight server.
- Retrieving the Query Results:
results = client.do_get(flight_info.endpoints[0].ticket, options)
The do_get()
function fetches the results of the query from the server. It takes in a ticket, which points to the data location, and the options to pass authentication headers.
- Reading and Printing Results:
print(results.read_all())
Finally, the read_all()
function is called to read all of the results into memory, and print()
displays the data.
Benefits of Using Apache Arrow Flight
- High Performance: Arrow Flight is optimized for fast, high-volume data transfers, making it ideal for large datasets.
- gRPC Communication: The use of gRPC allows for more efficient, low-latency communication between systems.
- Cross-Language Support: Arrow Flight works across multiple programming languages, providing flexibility in how data is accessed and processed.
Summary
Apache Arrow Flight with PyArrow offers an efficient and powerful way to transport data between systems, especially in high-performance environments. Using the example above, you can easily connect to Dremio, execute queries, and retrieve data in a highly optimized fashion. The combination of Arrow’s in-memory data structures and Flight’s fast data transport capabilities makes it an excellent tool for scalable, real-time data analytics.
Conclusion
In this blog, we explored the powerful capabilities of PyArrow for data analytics and efficient data handling. We began by setting up a practice environment using a Python Data Science Notebook Docker Image, which provides a comprehensive suite of pre-installed libraries for data manipulation and analysis.
We discussed the core benefits of PyArrow over traditional libraries like Pandas, focusing on its performance advantages, particularly for large datasets. PyArrow’s columnar memory layout and efficient in-memory processing make it a go-to tool for high-performance analytics.
Throughout the blog, we covered key PyArrow objects like Table
, RecordBatch
, Array
, Schema
, and ChunkedArray
, explaining how they work together to enable efficient data processing. We also demonstrated how to read and write Parquet, JSON, CSV, and Feather files, showcasing PyArrow’s versatility across various file formats commonly used in data science.
Additionally, we delved into essential data operations like filtering, joining, and aggregating data using PyArrow. These operations allow users to handle large datasets efficiently while performing complex analyses with minimal memory usage.
Lastly, we introduced Apache Arrow Flight as a high-performance transport layer for data transfer. We provided a detailed example of how to connect to Dremio, execute SQL queries, and retrieve results using Arrow Flight, highlighting its benefits for scalable, real-time data access.
With these tools and techniques, you are equipped to perform efficient data analytics using PyArrow, whether you’re working with local files or connecting to powerful cloud-based platforms like Dremio. By leveraging PyArrow’s capabilities, you can handle big data tasks with speed and precision, making it an indispensable tool for modern